概述
1 简介
适配器(Adapter)模式可以将一个类的接口和另一个类的接口匹配起来,使得原本由于接口不兼容而不能一起工作的那些类可以一起工作。使用的前提是不能或不想修改原来的适配者(Adaptee)接口和抽象目标类(Target)接口。如向第三方购买了一些类、控件,如果没有原代码,这时使用适配器模式可以统一对象访问接口。适配器包含类适配器和对象适配器。
- 类适配器:适配器类(Adapter)继承自适配者类(Adaptee)
- 对象适配器:适配器类(Adapter)聚合了适配者类(Adaptee)
适配器模式更多的是强调对代码的组织,而不是功能的实现。
使用场景:适配者类(Adaptee)没有 request() 方法,而客户期待这个方法,但在适配者类(Adaptee)中实现了specificRequest() 方法,该方法所提供的实现正是客户所需要的。为了使客户能够使用适配者类(Adaptee),提供了一个中间类,即适配器类(Adapter)。
2 模式
2.1 类适配器
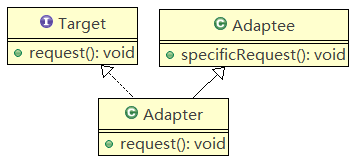
public class Adapter extends Adaptee implements Target{
@Override
public void request() {
specificRequest();
}
}
2.2 对象适配器
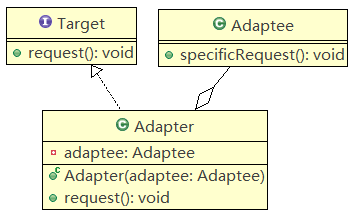
public class Adapter implements Target{
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee=adaptee;
}
public void request() {
adaptee.specificRequest();
}
}
3 案例分析
设计一个可以模拟各种动物行为的机器人,在机器人中定义了一系列方法,如机器人叫喊方法 cry() 、机器人移动方法 move() 等。如果希望在不修改已有代码的基础上使得机器人能够模拟各种动物叫(cry)、移动(move),可以使用适配器模式进行系统设计。
(1)目标类(Robot)
public interface Robot {
public void cry();
public void move();
}
(2)适配者类(Dog、Bird)
public class Dog {
public void wang() {
System.out.println("汪汪汪");
}
public void run() {
System.out.println("狗在狂奔");
}
}
public class Bird {
public void tweedle() {
System.out.println("叽叽叽");
}
public void fly() {
System.out.println("鸟儿在飞舞");
}
}
(3)适配器类(DogAdapter)
类适配器:
public class DogAdapter extends Dog implements Robot{
@Override
public void cry() {
wang();
}
@Override
public void move() {
run();
}
}
public class BirdAdapter extends Bird implements Robot{
@Override
public void cry() {
tweedle();
}
@Override
public void move() {
fly();
}
}
对象适配器:
public class DogAdapter implements Robot{
public Dog dog;
public DogAdapter(Dog dog) {
this.dog=dog;
}
@Override
public void cry() {
dog.wang();
}
@Override
public void move() {
dog.run();
}
}
public class BirdAdapter implements Robot{
public Bird bird;
public BirdAdapter(Bird bird) {
this.bird=bird;
}
@Override
public void cry() {
bird.tweedle();
}
@Override
public void move() {
bird.fly();
}
}
(4)客户端(Client)
public class Client {
public static void main(String[] args) {
//类适配器
Robot robot=new DogAdapter();
robot.cry();
robot.move();
Robot robot2=new BirdAdapter();
robot2.cry();
robot2.move();
//对象适配器
// Robot robot=new DogAdapter(new Dog());
// robot.cry();
// robot.move();
// Robot robot2=new BirdAdapter(new Bird());
// robot2.cry();
// robot2.move();
}
}
4 拓展延伸——缺省适配器模式
当不需要全部实现接口提供的方法时,可先设计一个抽象类实现该接口,并为接口中的每个方法提供一个默认实现(空方法),那么该抽象类的子类可有选择的覆盖父类的某些方法来实现需求。
(1)适配者接口(Service)
public interface Service {
public void method1();
public void method2();
public void method3();
}
(2)缺省适配器类(AbstractService)
使用空方法(也称钩子方法,Hook Method)的形式实现了在接口中声明的方法。
public class AbstractService implements Service{
@Override
public void method1() { }
@Override
public void method2() { }
@Override
public void method3() { }
}
(3)具体业务类
只选择使用 method1() 和 method3()
public class ConcreteService extends AbstractService{
@Override
public void method1() {
System.out.println("具体业务方法一");
}
@Override
public void method3() {
System.out.println("具体业务方法三");
}
}
在 JDK 类库的事件处理包 java.awt.event 中广泛使用了缺省适配器模式,如 WindowAdapter、KeyAdapter、MouseAdapter 等。如果实现窗口事件,可以通过实现 WindowListener 接口,或继承 WindowAdapter 类。前者需要实现接口中定义的7个方法,后者只需覆盖类中需要使用的方法。
最后
以上就是喜悦发卡为你收集整理的【设计模式】适配器模式的全部内容,希望文章能够帮你解决【设计模式】适配器模式所遇到的程序开发问题。
如果觉得靠谱客网站的内容还不错,欢迎将靠谱客网站推荐给程序员好友。
发表评论 取消回复