概述
原代码
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:800px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
flex-grow flex-shrink flex-basis flex都用在子元素上
flex-grow 项目的放大比例---前提有剩余空间
项目的放大比例,值为0时,不放大。
值大于0,值为几就放大几倍
这个放大的值来源于div规定完宽度后剩下的值进行平均分。
比如:原代码宽为800,3个子div宽度之和为300,还剩下500的宽度.这500就是剩余空间
案例1 父元素宽600
图一图二图三都是一个图,这样做是为把每个子div的宽度截下来
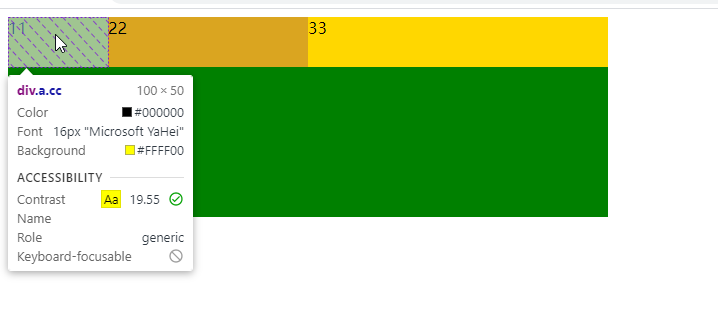

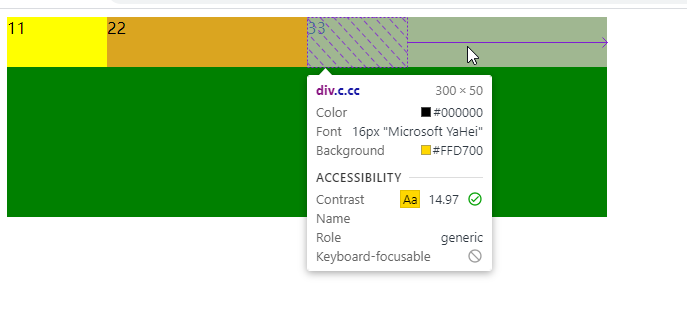
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:600px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
/* 值为0时,不放大 放大0倍 100+100*0 */
flex-grow: 0;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
/* 放大1倍 100+100*1 */
flex-grow: 1;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
/* 放大2倍 100+100*2 */
flex-grow: 2;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
案例二 父元素宽为800
图一图二图三都是一个图,这样做是为把每个div的宽度截下来



<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:800px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-grow: 0;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-grow: 1;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-grow: 2;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
案列3 宽为800 flex-grow有一个值为0.x
这个算法 (800-100-100-100)/(2+1+0.2)=156.25
156.25*0.2=31.25
156.25*1=156.25
156.25*2=312.5
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:800px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-grow: 0.2;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-grow: 1;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-grow: 2;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
案列4 当flex-grow总值小于1时
800-300=500
5000*0.2=100
500*0.3=150
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:800px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-grow: 0.2;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-grow: 0.3;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-grow: 0.3;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
flex-shrink的缩小比列----前提空间不够
案列1 值相加之和 大于1
300-250=50px -----少了50px
1+2+3=6
50/6~=8.33
100-8.33=91.76
100-(8.333*2)=83.33------8.333*2 值保留2位 16.666----16.67
100-(8.33*3)=75
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-shrink: 1;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-shrink: 2;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-shrink: 3;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
案列2 值相加之和 等于1 里面还是要分配
100-50*0.4=80
100-50*0.2=90
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-shrink: 0.4;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-shrink: 0.4;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-shrink: 0.2;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
案列3 每个子div值等于1 平分
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-shrink: 1;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-shrink: 1;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-shrink: 1;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
案列4 每个div值和相加小于1 增长
80+95+90=265
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-shrink: 0.4;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-shrink: 0.1;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-shrink: 0.2;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
flex-basic 伸缩基准值
flex-basic值为auto时,意思就是flex-basic的值等于子元素本身的宽度/高度。
当方向为row时
这个的作用和width一样,并且优先级高于width。等于只要写了flex-basic,子元素的宽度就是flex-basic的值。----这个是当总值<父divwidth
row应该和column一样,以后再说。
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-basis: 80px;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
/* 现在宽就为 width:80 */
flex-basis: 80px;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-basis: 80px;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
当方向为column
这个的作用和height一样,并且优先级高于height。等于只要写了flex-basic,子元素的高度就是flex-basic的值。------总值小于父div的height
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
flex-direction: column;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-basis: 60px;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-basis: 60px;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-basis: 60px;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
当子元素div的高度之和大于父元素,这个时候的flex-basic值无效,页面效果是几个根据比列平分父元素的高值。----总值大于父div的height
200/3~=66.67
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
flex-direction: column;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-basis: 80px;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-basis: 80px;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-basis: 80px;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
不想算了,以后再说
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
width:250px;
height: 200px;
background-color: #008000;
display: flex;
flex-direction: column;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex-basis: 50px;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex-basis: 100px;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex-basis: 80px;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
flex语法糖
flex属性是 flex-grow flex-shrink flex-basis 的简写,默认值为0 1 auto
auto 意思为 1 1 auto 多余空间 和少了空间都会自动平分
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
/* 200px 400px 代表了多余空间 和少的空间 */
/* width:400px; */
width:200px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex: auto;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex:auto;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex: auto;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
initial 意思为 0 1 auto 剩余空间不管 少的空间管
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
/* 200px 400px 代表了多余空间 和少的空间 */
/* width:400px; */
width:200px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex: initial;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex:initial;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex: initial;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
none 意思为 0 0 auto 剩余空间 少的空间 都不管
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.container{
/* 200px 400px 代表了多余空间 和少的空间 */
/* width:400px; */
width:200px;
height: 200px;
background-color: #008000;
display: flex;
}
.a{
width: 100px;
height: 50px;
background-color:yellow;
flex:none;
}
.b{
width: 100px;
height: 50px;
background-color:goldenrod;
flex:none;
}
.c{
width: 100px;
height: 50px;
background-color:gold;
flex: none;
}
</style>
</head>
<body>
<div class="container">
<div class="a cc">11</div>
<div class="b cc">22</div>
<div class="c cc">33</div>
</div>
</body>
</html>
最后
以上就是威武大象为你收集整理的弹性盒子----思路分析 flex-grow flex-shrink flex-basis flexflex-grow flex-shrink flex-basis flex都用在子元素上flex-grow 项目的放大比例---前提有剩余空间flex-shrink的缩小比列----前提空间不够flex-basic 伸缩基准值flex语法糖的全部内容,希望文章能够帮你解决弹性盒子----思路分析 flex-grow flex-shrink flex-basis flexflex-grow flex-shrink flex-basis flex都用在子元素上flex-grow 项目的放大比例---前提有剩余空间flex-shrink的缩小比列----前提空间不够flex-basic 伸缩基准值flex语法糖所遇到的程序开发问题。
如果觉得靠谱客网站的内容还不错,欢迎将靠谱客网站推荐给程序员好友。
发表评论 取消回复