概述
目标:实现查询用户列表
整体架构图
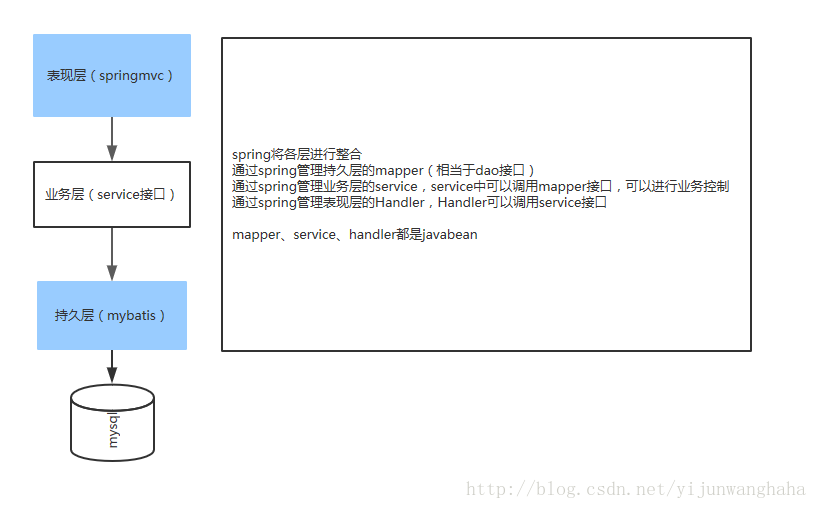
1.整合dao层
mybatis和spring整合,通过spring管理mapper接口
使用mapper的扫描器自动扫描mapper接口在spring中进行注册
2.整理service层
通过spring管理service接口
使用配置方式将service接口配置在spring配置文件中
实现事务控制
3.整合springmvc
由于springmvc是spring的模块,不需要整合。
环境
所需要的jar包:
数据库驱动包:mysql
mybatis的jar包
mybatis和spring整合包
log4j包
dbcp数据库连接池包
spring4.0所有jar包
jstl包
数据库结构
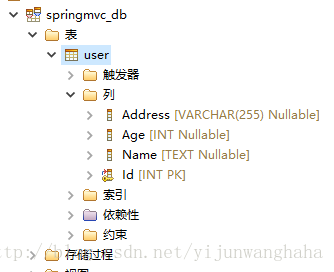
工程结构
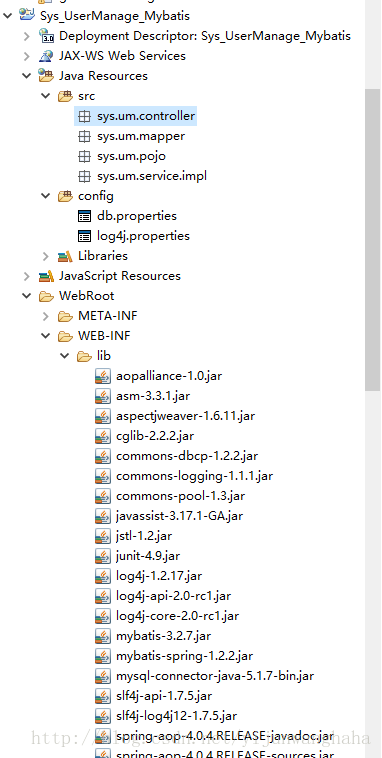
db.properties
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/users
jdbc.username=root
jdbc.password=
log4j.properties
# Global logging configurationuFF0Cu5EFAu8BAEu5F00u53D1u73AFu5883u4E2Du8981u7528debug
log4j.rootLogger=DEBUG, stdout
# Console output...
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%5p [%t] - %m%n
整合Dao
mybatis需要的配置文件
sqlMapConfig.xml(mybatis自己的配置文件,加载mapper文件)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- 配置别名 -->
<typeAliases>
<!--批量扫描别名-->
<package name="sys.um.pojo" />
</typeAliases>
<!-- 配置mapper,使用自动扫描器时,mapper.xml文件如果和mapper.java接口在一个目录则此处不用定义mappers -->
<mappers>
<package name="sys.um.mapper" />
</mappers>
</configuration>
mybatis和spring整合的配置文件
applicationContext-dao.xml
配置:
数据源
SqlSessionFactory
mapper扫描器
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.0.xsd ">
<!-- 加载配置文件 -->
<!-- 加载db.properties文件中的内容,db.properties中的key命名统一的规则 -->
<context:property-placeholder location="classpath:db.properties" />
<!-- 配置数据源,dbcp -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource"
destroy-method="close">
<property name="driverClassName" value="${jdbc.driver}" />
<property name="url" value="${jdbc.url}" />
<property name="username" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
<property name="maxActive" value="30" />
<property name="maxIdle" value="5" />
</bean>
<!-- 让spring管理sqlsessionfactory 使用mybatis和spring整合包中的 -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<!-- 数据库连接池 -->
<property name="dataSource" ref="dataSource" />
<!-- 加载mybatis的全局配置文件 -->
<property name="configLocation" value="classpath:mybatis/sqlMapConfig.xml" />
</bean>
<!-- mapper扫描器 -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<!--扫描包路径,如果需要扫描多个包,中间使用半角逗号隔开-->
<property name="basePackage" value="sys.um.mapper"></property>
<property name="sqlSessionFactoryBeanName" value="sqlSessionFactory" />
</bean>
</beans>
逆向工程生成po类及mapper(单表的增删改查)
手动定义mapper(针对综合查询mapper,一般情况会有关联查询,建议自定义mapper)
UserMapperCustom.xml
<mapper namespace="sys.um.mapper.UserMapperCustom">
<!-- 定义用户查询sql片段,就是用户查询条件 -->
<sql id="query_user_where">
<!-- 使用动态条件,通过if判断,满足条件进行sql拼接 -->
<!-- 用户的查询条件是要通过UserQueryVo包装对象中UserCustom属性传递 -->
<if test="userCustom!=null">
<if test="userCustom.name!=null and userCustom.name!=''">
user.Name like "%${userCustom.name}%"
</if>
</if>
</sql>
<!-- 用户列表查询 -->
<!-- parameterType传入包装对象(pojo的包装) -->
<!-- resultType建议使用扩展对象 -->
<select id="findUsersList" parameterType="sys.um.pojo.UsersQueryVo"
resultType="sys.um.pojo.UserCustom">
select * from user
<where>
<include refid="query_user_where"></include>
</where>
</select>
</mapper>
UserMapperCustom.java
public interface UserMapperCustom {
// 用户查询列表
public List<UserCustom> findUsersList(UsersQueryVo usersQueryVo) throws Exception;
}
UsersQueryVo.java(包装对象)
/*
* 包装对象
* */
public class UsersQueryVo {
// 用户信息
private User user;
// 为了系统可扩展性,对原始生成的po进行扩展
private UserCustom userCustom;
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
public UserCustom getUserCustom() {
return userCustom;
}
public void setUserCustom(UserCustom userCustom) {
this.userCustom = userCustom;
}
}
整合service
让spring管理service接口
定义service接口
public interface UsersService {
public List<UserCustom> findUsersList(UsersQueryVo usersQueryVo) throws Exception;
}
实现接口实现类(Impl)
public class UsersServiceImpl implements UsersService {
@Autowired
private UserMapperCustom userMapperCustom;
@Override
public List<UserCustom> findUsersList(UsersQueryVo usersQueryVo) throws Exception {
// 通过UserMapperCustom查询数据库
return userMapperCustom.findUsersList(usersQueryVo);
}
}
在spring容器配置service
创建applicationContext-service.xml,文件中配置service
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.2.xsd ">
<!-- 用户管理的service -->
<bean id="userService" class="sys.um.service.impl.UsersServiceImpl" />
</beans>
事务控制applicationContext-transcation.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.2.xsd ">
<!-- 事务管理器 对mybatis操作数据库的事物控制:spring使用jdbc的事务控制类 -->
<bean id="transactionManager"
class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<!-- 数据源
dataSource 在applicationContext-dao.xml里配置 -->
<property name="dataSource" ref="dataSource" />
</bean>
<!-- 通知 -->
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes>
<!-- 传播行为
函数名以以下开头的必须实现事物(这样写为了规范开发)-->
<tx:method name="save*" propagation="REQUIRED" />
<tx:method name="delete*" propagation="REQUIRED" />
<tx:method name="insert*" propagation="REQUIRED" />
<tx:method name="update*" propagation="REQUIRED" />
<tx:method name="find*" propagation="SUPPORTS" read-only="true" />
</tx:attributes>
</tx:advice>
<!--调用通知-->
<aop:config>
<aop:advisor advice-ref="txAdvice"
pointcut="execution(* sys.um.service.impl.*.*(..))" />
</aop:config>
</beans>
整合springmvc
创建springmvc.xml文件,配置处理器映射器,适配器,视图解析器
配置springmvc文件
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.2.xsd ">
<!-- 可以扫描controller、service... 这里让扫描controller,指定Controller的包 -->
<context:component-scan base-package="sys.um.controller" />
<!-- 使用mvc:annotation-driven(mvc注解驱动)代替上班注解映射器和注解适配器配置 mvc:annotation-driven默认加载很多参数绑定方法,比如json转换解析器,实际开发使用此配置 -->
<mvc:annotation-driven></mvc:annotation-driven>
<!-- 视图解析器 -->
<!-- 解析jsp,默认使用jstl标签,classpath下得有jstl的包 -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="WEB-INF/jsp/" />
<property name="suffix" value=".jsp" />
</bean>
</beans>
配置前端控制器
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>Sys_UserManage_Mybatis</display-name>
<!-- 加载spring容器 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/classes/spring/applicationContext-*.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!-- springmvc前端控制器 -->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<!-- contextConfigLocation配置springmvc加载的配置文件(配置处理器、映射器等) -->
<!-- 如果不配置contextConfigeLocation,默认加载的是/WEB-INF/servlet名称-servlet.xml(springmvc-servlet.xml) -->
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring/springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<!-- 第一种:*.action,访问以.action结尾由DispatcherServlet进行解析 -->
<!-- 第二种:/,所有访问的地址都由DispatcherServlet进行解析,对于静态文件的解析需要配置不让DispatcherServlet进行解析
(使用此种方式可以实现RESTful风格url) -->
<url-pattern>*.action</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
</web-app>
编写controller
@Controller
public class UsersController {
//用户查询
@Autowired
private UsersService usersService;
@RequestMapping("/queryUsers")
public ModelAndView queryUsers() throws Exception {
List<UserCustom> usersList=usersService.findUsersList(null);
ModelAndView modelAndView = new ModelAndView();
// 填充对象
modelAndView.addObject("userList", usersList);
// 指定视图
modelAndView.setViewName("user");
return modelAndView;
}
}
编写jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
<table>
<tr>
<td>name</td>
</tr>
<c:forEach items="${userList}" var="item">
<tr>
<td>${item.name}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
加载spring容器
将mapper、service、controller加载到spring容器中(将applicationContext-dao、service、transcation.xml加载到容器当中)
在web.xml中,使用通配符加载上边的配置文件
<!-- 加载spring容器 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/classes/spring/applicationContext-*.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
完成
最后
以上就是单纯冬日为你收集整理的springmvc和mybatis整合-查询用户列表的全部内容,希望文章能够帮你解决springmvc和mybatis整合-查询用户列表所遇到的程序开发问题。
如果觉得靠谱客网站的内容还不错,欢迎将靠谱客网站推荐给程序员好友。
本图文内容来源于网友提供,作为学习参考使用,或来自网络收集整理,版权属于原作者所有。
发表评论 取消回复