概述
一:缓存的概念
1.1. 什么是缓存(cache)
1) cache是高速缓冲存储器,主要解决频繁使用的数据快速访问的问题。
2) 如果两个硬件或者软件之间的速度存在较大差异,主要使用缓存协调两者的速度差异
1.2. 缓存的分类
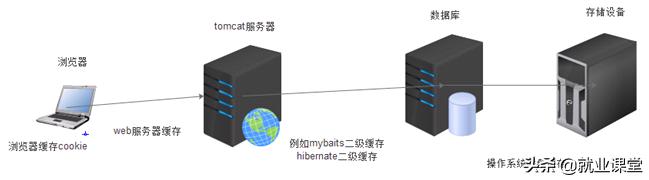
1) 操作系统磁盘缓存:减少磁盘机械操作。
2) 数据库缓存:减少应用程序对数据库服务器的IO操作。
3) web服务器缓存:减轻web服务器的压力。
4) 浏览器缓存:访问速度快,提升用户体验度,减轻网站压力。
二.redis(单机版)实现mybatis的二级缓存
2.1. 环境准备
使用maven搭建一套ssm框架,并创建测试类查询emp表。测试代码如下:
/**
* 不使用缓存的查询时间
*/
public static void main(String[] args) {
ApplicationContext ac =new ClassPathXmlApplicationContext("applicationContext.xml");
EmpMapper empMapper=(EmpMapper) ac.getBean("empMapper");
Long begin=System.currentTimeMillis();
empMapper.findAllEmp();
Long end=System.currentTimeMillis();
System.out.println("花费时间:"+(end-begin));
}
结果截图:

2.2. 配置mybais的二级缓存
1) 修改配置文件mapper.xml
加上,
2) 修改配置文件applicationContext.xml,开启mybatis缓存,在
"configurationProperties">
"cacheEnabled">true
"lazyLoadingEnabled">false
"aggressiveLazyLoading">true
"multipleResultSetsEnabled">true
"useColumnLabel">true
"useGeneratedKeys">true
"autoMappingBehavior">FULL
"defaultExecutorType">BATCH
"defaultStatementTimeout">25000
3) 添加配置文件spring-redis.xml
"poolConfig" class="redis.clients.jedis.JedisPoolConfig">
"maxIdle" value="2000" />
"maxTotal" value="20000" />
"minEvictableIdleTimeMillis" value="300000">
"numTestsPerEvictionRun" value="3">
"timeBetweenEvictionRunsMillis" value="60000">
"maxWaitMillis" value="20000" />
"testOnBorrow" value="false" />
"jedisConnectionFactory" class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory">
"hostName" value="192.168.115.164" />
"port" value="6379" />
"poolConfig" ref="poolConfig" />
"redisCache" class="com.aaa.util.RedisCacheTransfer">
"jedisConnectionFactory" ref="jedisConnectionFactory">
4) 创建mybatis cache接口的实现类RedisCache
/**
* @author sunshaoshan
* @description 使用第三方缓存服务器,处理二级缓存
* @company AAA软件
* 2017-6-29下午2:16:56
*/
public class RedisCache implements Cache {
private static JedisConnectionFactory jedisConnectionFactory;
private final String id;
private final ReadWriteLock readWriteLock = new ReentrantReadWriteLock();
public RedisCache(final String id){
if (id == null) {
throw new IllegalArgumentException("cache instances require an ID");
}
this.id = id;
}
/**
* @description: 清空redis缓存
* @author
* @param
* @return
*/
@Override
public void clear() {
RedisConnection connection = null;
try {
connection = jedisConnectionFactory.getConnection();
connection.flushDb();//清空redis中的数据
connection.flushAll();//#移除所有key从所有库中
} catch (Exception e) {
e.printStackTrace();
}finally{
if (connection != null) {
connection.close();
}
}
}
@Override
public String getId() {
return this.id;
}
/**
* @description: 根据key获取redis缓存中的值
* @author
* @param
* @return
*/
@Override
public Object getObject(Object key) {
System.out.println("--------------------------------key:["+key+"]");
Object result = null;
RedisConnection connection = null;
try {
connection = jedisConnectionFactory.getConnection();
RedisSerializer serializer = new JdkSerializationRedisSerializer();
//serializer.serialize(key)将key序列化
//connection.get(serializer.serialize(key))根据key去redis中获取value
//serializer.deserialize将value反序列化
result = serializer.deserialize(connection.get(serializer.serialize(key)));
} catch (Exception e) {
e.printStackTrace();
}finally{
if (connection != null) {
connection.close();
}
}
return result;
}
@Override
public ReadWriteLock getReadWriteLock() {
return this.readWriteLock;
}
@Override
public int getSize() {
int result = 0;
RedisConnection connection = null;
try {
connection = jedisConnectionFactory.getConnection();
result = Integer.valueOf(connection.dbSize().toString());
} catch (Exception e) {
e.printStackTrace();
}finally{
if (connection != null) {
connection.close();
}
}
return result;
}
/**
* @description: 将数据保存到redis缓存
* @author
* @param
* @return
*/
@Override
public void putObject(Object key, Object value) {
System.out.println(">>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>key:"+key);
RedisConnection connection = null;
try {
connection = jedisConnectionFactory.getConnection();
RedisSerializer serializer = new JdkSerializationRedisSerializer();
System.out.println("**"+serializer.serialize(key));
//serializer.serialize(value)将value序列化,serializer.serialize(key)将key序列化
connection.set(serializer.serialize(key), serializer.serialize(value));
} catch (Exception e) {
e.printStackTrace();
}finally{
if (connection != null) {
connection.close();
}
}
}
/**
* @description: 根据key清除redis缓存中对应 的值
* @author
* @param
* @return
*/
@Override
public Object removeObject(Object key) {
RedisConnection connection = null;
Object result = null;
try {
connection = jedisConnectionFactory.getConnection();
RedisSerializer serializer = new JdkSerializationRedisSerializer();
result = connection.expireAt(serializer.serialize(key), 0);
} catch (Exception e) {
e.printStackTrace();
}finally{
if (connection != null) {
connection.close();
}
}
return result;
}
public static void setJedisConnectionFactory(JedisConnectionFactory jedisConnectionFactory) {
RedisCache.jedisConnectionFactory = jedisConnectionFactory;
}
5) 创建中间类,解决RedisCache.jedisConnectionFactory的静态注入,从而使MyBatis实现第三方缓存
/**
* @author sunshaoshan
* @description 创建中间类RedisCacheTransfer,完成RedisCache.jedisConnectionFactory的静态注入
* @company AAA软件
* 2018-1-12上午8:46:18
*/
public class RedisCacheTransfer {
public void setJedisConnectionFactory(JedisConnectionFactory jedisConnectionFactory) {
RedisCache.setJedisConnectionFactory(jedisConnectionFactory);
}
}
6) 测试类
/**
* 使用缓存的查询时间
*/
public static void main(String[] args) {
ApplicationContext ac =new ClassPathXmlApplicationContext("applicationContext.xml","spring-redis.xml");
EmpMapper empMapper=(EmpMapper) ac.getBean("empMapper");
Long begin=System.currentTimeMillis();
List empList = empMapper.findAllEmp();
System.out.println("员工数:"+empList.size());
Long end=System.currentTimeMillis();
System.out.println("花费时间:"+(end-begin));
}
7) 测试效果
第一次查询 redis服务器没有缓存,所以所耗时间较长

第二次查询

三:redis(集群版)实现mybatis的二级缓存
3.1. 修改配置文件spring-redis.xml
"redisClusterConfiguration" class="org.springframework.data.redis.connection.RedisClusterConfiguration">
"maxRedirects" value="3">
"clusterNodes">
"org.springframework.data.redis.connection.RedisClusterNode">
"0" value="192.168.115.164">
"1" value="7001">
"org.springframework.data.redis.connection.RedisClusterNode">
"0" value="192.168.115.164">
"1" value="7002">
"org.springframework.data.redis.connection.RedisClusterNode">
"0" value="192.168.115.164">
"1" value="7003">
"org.springframework.data.redis.connection.RedisClusterNode">
"0" value="192.168.115.164">
"1" value="7004">
"org.springframework.data.redis.connection.RedisClusterNode">
"0" value="192.168.115.164">
"1" value="7005">
"org.springframework.data.redis.connection.RedisClusterNode">
"0" value="192.168.115.164">
"1" value="7006">
"jedisConnectionFactory" class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory">
"poolConfig" ref="poolConfig"/>
"clusterConfig" ref="redisClusterConfiguration"/>
3.2. 测试效果
1) 启动redis集群
redis-server /usr/redis_cluster/7001/redis.conf
redis-server /usr/redis_cluster/7002/redis.conf
redis-server /usr/redis_cluster/7003/redis.conf
redis-server /usr/redis_cluster/7004/redis.conf
redis-server /usr/redis_cluster/7005/redis.conf
redis-server /usr/redis_cluster/7006/redis.conf
2) 清空集群中的所有key,要在主节点上操作
使用FLUSHALL命令在所有的主节点
3) 第一次查询 redis所有的节点为空,所以查询效率慢

4) 第二次查询 redis有缓存,所以查询效率快一倍

最后
以上就是内向毛巾为你收集整理的redis 清空缓存_Redis缓存的全部内容,希望文章能够帮你解决redis 清空缓存_Redis缓存所遇到的程序开发问题。
如果觉得靠谱客网站的内容还不错,欢迎将靠谱客网站推荐给程序员好友。
发表评论 取消回复