我是靠谱客的博主 长情曲奇,最近开发中收集的这篇文章主要介绍QT 自带数据库使用 数据库查询结果显示到ui窗口 学习面向对象语言与SQL SEREVR数据库的连接方法及嵌入式SQL语言查询编程,觉得挺不错的,现在分享给大家,希望可以做个参考。
概述
一、实验目的
- 本次实验的主要目的是主高级语句的使用,学习主高级语言与数据库的连接方法与编程技巧。
- 嵌入式SQL语言与主语言的联合编程。
二、实验要求
1.要求学生独立完成实验内容,画出E-R图及程序功能图;
2.按照实验步骤完成实验后,撰写报告内容,并对操作结果进行截图,写出主要关键程序代码。
三、实验内容及实验结果与主要代码
1.学习主语言与数据库的连接方法,写出数据库的连接语句。
2.采用嵌入SQL语言用游标实现如下界面功能的查询。学会使用嵌入SQL对数据库进行单表精确查询、模糊查询的方法
建立学生表:S(SNO,SNAME,SSEX,SAGE,SADDR)
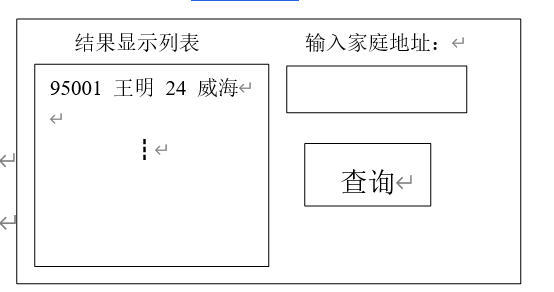
项目结构
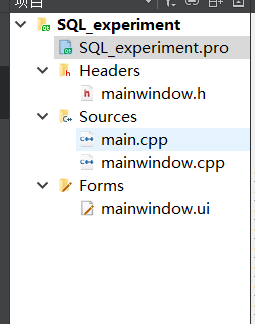
详细说明在代码里
pro文件:
#-------------------------------------------------
#
# Project created by QtCreator 2021-06-24T14:46:14
#
#-------------------------------------------------
QT += core gui
QT += sql
# 这里是添加了qt+=sql,可以使用数据库了,qt5以上是直接自带sqlite数据库的。
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
TARGET = SQL_experiment
TEMPLATE = app
# The following define makes your compiler emit warnings if you use
# any feature of Qt which as been marked as deprecated (the exact warnings
# depend on your compiler). Please consult the documentation of the
# deprecated API in order to know how to port your code away from it.
DEFINES += QT_DEPRECATED_WARNINGS
# You can also make your code fail to compile if you use deprecated APIs.
# In order to do so, uncomment the following line.
# You can also select to disable deprecated APIs only up to a certain version of Qt.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
SOURCES +=
main.cpp
mainwindow.cpp
HEADERS +=
mainwindow.h
FORMS +=
mainwindow.ui
.h文件
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private slots:
void on_AddrIn_destroyed();
void on_pushButton_clicked();
private:
Ui::MainWindow *ui;
};
#endif // MAINWINDOW_H
main.cpp
#include "mainwindow.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
mainwindow.cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <qdebug.h>
#include <QSqlDatabase>
#include <QSqlError>
#include <QSqlQuery>
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
//这里是mainwindow的一开始就会运行的代码,把数据库开启过程放在这就不用每次点击按钮之后才发生数据库的创建了。
ui->setupUi(this);
//建立并打开数据库
QSqlDatabase database;
database = QSqlDatabase::addDatabase("QSQLITE");
database.setDatabaseName("MyDataBase.db");
if (!database.open())
{
qDebug() << "Error: Failed to connect database." << database.lastError();
}
else
{
qDebug() << "Succeed to connect database." ;
}
//申明对象
QSqlQuery sql_query;
//先删除数据库中的表,避免后面每次进入都会重复创建表格导致创建表格报错 Fail to create table. QSqlError("1", "Unable to execute statement", "table S already exists")
if(!sql_query.exec("drop table S"))
{
qDebug() << "Error: Fail to drop table."<< sql_query.lastError();
}
else
{
qDebug() << "Table dropped!";
}
//创建表格,每次进入window都会运行,所以其实运行一次之后就可以不再运行了的。
if(!sql_query.exec("create table S(sno int primary key, sname char(10),ssex char(2), sage int , saddr char(50))"))
{
qDebug() << "Error: Fail to create table."<< sql_query.lastError();
}
else
{
qDebug() << "Table created!";
}
//插入数据
if(!sql_query.exec("INSERT INTO S VALUES(95001, "王明", "男",24,"威海"),(95002,"小飞","女", 24,"衡阳")"))
{
qDebug() << sql_query.lastError();
}
else
{
qDebug() << "inserted successful!";
}
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_AddrIn_destroyed()
{
}
void MainWindow::on_pushButton_clicked()
{
//得到ui中名字为AddrIn的组件的内容,也就是输入框中的内容,注意:在构建ui窗口的时候,输入框要选择input widget中的组件。
QString addr=ui->AddrIn->toPlainText();
QString sno;
QString sname;
QString ssex;
QString sage;
QString saddr;
QSqlQuery sql_query;
QString str = QString("select *from s where saddr like '%1'").arg(addr);
// sql_query.exec("select {home} from S");
sql_query.exec(str);
if(!sql_query.exec())
{
qDebug()<<sql_query.lastError();
}
else
{
while(sql_query.next())
{
sno = sql_query.value(0).toString();
sname = sql_query.value(1).toString();
ssex = sql_query.value(2).toString();
sage = sql_query.value(3).toString();
saddr = sql_query.value(4).toString();
//将数据写入到ui组件ResultView中,如果你的setText报错'class QListView' has no member named 'setText' ui->ResultView->setText('1')
//,那么可能是你在选择组件的时候出错了,可以看看我的博客:https://blog.csdn.net/jklw4/article/details/118194604
ui->ResultView->setText(sno+" "+sname+" "+ssex+" "+sage+" "+saddr+" "+"n");
}
}
}
mainwindow.ui
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>MainWindow</class>
<widget class="QMainWindow" name="MainWindow">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>577</width>
<height>388</height>
</rect>
</property>
<property name="windowTitle">
<string>MainWindow</string>
</property>
<widget class="QWidget" name="centralWidget">
<widget class="QLabel" name="label">
<property name="geometry">
<rect>
<x>350</x>
<y>90</y>
<width>111</width>
<height>31</height>
</rect>
</property>
<property name="text">
<string>输入家庭地址:</string>
</property>
</widget>
<widget class="QLabel" name="label_2">
<property name="geometry">
<rect>
<x>140</x>
<y>60</y>
<width>91</width>
<height>21</height>
</rect>
</property>
<property name="text">
<string>查询结果显示</string>
</property>
</widget>
<widget class="QPushButton" name="pushButton">
<property name="geometry">
<rect>
<x>370</x>
<y>210</y>
<width>91</width>
<height>61</height>
</rect>
</property>
<property name="text">
<string>查询</string>
</property>
</widget>
<widget class="QTextBrowser" name="ResultView">
<property name="geometry">
<rect>
<x>50</x>
<y>100</y>
<width>256</width>
<height>192</height>
</rect>
</property>
</widget>
<widget class="QTextEdit" name="AddrIn">
<property name="geometry">
<rect>
<x>340</x>
<y>130</y>
<width>161</width>
<height>51</height>
</rect>
</property>
</widget>
</widget>
<widget class="QMenuBar" name="menuBar">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>577</width>
<height>26</height>
</rect>
</property>
</widget>
<widget class="QToolBar" name="mainToolBar">
<attribute name="toolBarArea">
<enum>TopToolBarArea</enum>
</attribute>
<attribute name="toolBarBreak">
<bool>false</bool>
</attribute>
</widget>
<widget class="QStatusBar" name="statusBar"/>
</widget>
<layoutdefault spacing="6" margin="11"/>
<resources/>
<connections/>
</ui>
最后
以上就是长情曲奇为你收集整理的QT 自带数据库使用 数据库查询结果显示到ui窗口 学习面向对象语言与SQL SEREVR数据库的连接方法及嵌入式SQL语言查询编程的全部内容,希望文章能够帮你解决QT 自带数据库使用 数据库查询结果显示到ui窗口 学习面向对象语言与SQL SEREVR数据库的连接方法及嵌入式SQL语言查询编程所遇到的程序开发问题。
如果觉得靠谱客网站的内容还不错,欢迎将靠谱客网站推荐给程序员好友。
本图文内容来源于网友提供,作为学习参考使用,或来自网络收集整理,版权属于原作者所有。
发表评论 取消回复