本教程操作环境:Windows7系统、react18版、Dell G3电脑。
一、React中的组件
react组件就是自己定义的非html标签,规定react组件首字母大写
:
class App extends Component{
}
<App />
登录后复制
二、父子组件
组件的相互调用中,把调用者
称为父组件,被调用者
称为子组件:
import React from 'react';
import Children from './Children';
class Up extends React.Component {
constructor(props){
super(props);
this.state = {
}
}
render(){
console.log("render");
return(
<div>
up
<Children />
</div>
)
}
}
export default Up;
登录后复制
import React from 'react';
class Children extends React.Component{
constructor(props){
super(props);
this.state = {
}
}
render(){
return (
<div>
Children
</div>
)
}
}
export default Children;
登录后复制
三、父组件给子组件传值
父组件向子组件传值使用props。父组件向子组件传值时,先将需要传递的值传递给子组件,然后在子组件中,使用props来接收父组件传递过来的值。
父组件在调用子组件的时候定义一个属性:
<Children msg="父组件传值给子组件" />
登录后复制
这个值msg
会绑定在子组件的props
属性上,子组件可以直接使用:
this.props.msg
登录后复制
父组件可以给组件传值,传方法,甚至可以把自己传递给子组件
3.1 传值
import React from 'react';
import Children from './Children';
class Up extends React.Component {
constructor(props){
super(props);
this.state = {
}
}
render(){
console.log("render");
return(
<div>
up
<Children msg="父组件传值给子组件" />
</div>
)
}
}
export default Up;
登录后复制
import React from 'react';
class Children extends React.Component{
constructor(props){
super(props);
this.state = {
}
}
render(){
return (
<div>
Children
<br />
{this.props.msg}
</div>
)
}
}
export default Children;
登录后复制
3.2 传方法
import React from 'react';
import Children from './Children';
class Up extends React.Component {
constructor(props){
super(props);
this.state = {
}
}
run = () => {
console.log("父组件run方法");
}
render(){
console.log("render");
return(
<div>
up
<Children run={this.run} />
</div>
)
}
}
export default Up;
登录后复制
import React from 'react';
class Children extends React.Component{
constructor(props){
super(props);
this.state = {
}
}
run = () => {
this.props.run();
}
render(){
return (
<div>
Children
<br />
<button onClick={this.run}>Run</button>
</div>
)
}
}
export default Children;
登录后复制
3.3 将父组件传给子组件
import React from 'react';
import Children from './Children';
class Up extends React.Component {
constructor(props){
super(props);
this.state = {
}
}
run = () => {
console.log("父组件run方法");
}
render(){
console.log("render");
return(
<div>
up
<Children msg={this}/>
</div>
)
}
}
export default Up;
登录后复制
import React from 'react';
class Children extends React.Component{
constructor(props){
super(props);
this.state = {
}
}
run = () => {
console.log(this.props.msg);
}
render(){
return (
<div>
Children
<br />
<button onClick={this.run}>Run</button>
</div>
)
}
}
export default Children;
登录后复制
四、子组件给父组件传值
子组件向父组件传值通过触发方法来传值
import React from 'react';
import Children from './Children';
class Up extends React.Component {
constructor(props){
super(props);
this.state = {
}
}
getChildrenData = (data) => {
console.log(data);
}
render(){
console.log("render");
return(
<div>
up
<Children upFun={this.getChildrenData}/>
</div>
)
}
}
export default Up;
登录后复制
import React from 'react';
class Children extends React.Component{
constructor(props){
super(props);
this.state = {
}
}
render(){
return (
<div>
Children
<br />
<button onClick={() => {this.props.upFun("子组件数据")}}>Run</button>
</div>
)
}
}
export default Children;
登录后复制
五、父组件中通过refs获取子组件属性和方法
import React from 'react';
import Children from './Children';
class Up extends React.Component {
constructor(props){
super(props);
this.state = {
}
}
clickButton = () => {
console.log(this.refs.children);
}
render(){
console.log("render");
return(
<div>
up
<Children ref="children" msg="test"/>
<button onClick={this.clickButton}>click</button>
</div>
)
}
}
export default Up;
```
```js
import React from 'react';
class Children extends React.Component{
constructor(props){
super(props);
this.state = {
title: "子组件"
}
}
runChildren = () => {
}
render(){
return (
<div>
Children
<br />
</div>
)
}
}
export default Children;
```
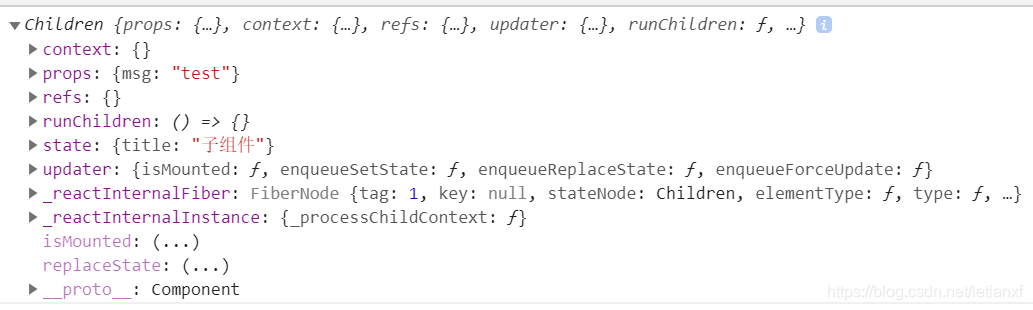
登录后复制
【相关推荐:Redis视频教程】
以上就是react中什么是父子组件的详细内容,更多请关注靠谱客其它相关文章!
最后
以上就是轻松小蚂蚁最近收集整理的关于react中什么是父子组件的全部内容,更多相关react中什么是父子组件内容请搜索靠谱客的其他文章。
本图文内容来源于网友提供,作为学习参考使用,或来自网络收集整理,版权属于原作者所有。
发表评论 取消回复