概述

本章节笔记源自
Java: The Complete Reference, Eleventh Edition, 11th Editionlearning.oreilly.com
这张内容太多了,我就选了看下来可能用到的记了下来。
java.lang
是自动Import进所有模块的。他包含了几乎一切java程序中的基础组件。Java: The Complete Reference, Eleventh Edition, 11th Editionjava.lang
是自动Import进所有模块的。他包含了几乎一切java程序中的基础组件。
java.lang
包含以下class

包含以下interfaces

Primitives 类型的Wrapper
抽象类Number
定义了了一个能够把byte,short,int这些原始类包装起来。同时number
也提供了方法能够把Object的值返回。比如doubleValue
返回值为double
,floatValue
的返回值为float
。
byte byteValue( )
double doubleValue( )
float floatValue( )
int intValue( )
long longValue( )
short shortValue( )
The values returned by these methods might be rounded, truncated, or result in a “garbage” value due to the effects of a narrowing conversion.
Numbers也提供了实际的subclasses来准确的保存各类数值型的值:Double,Float,Byte,Short,Integer, andLong.
其中,Double和Float分别是double和float的包装类。float的构建函数如下:
Float(double num)
Float(float num)
Float(String str) throws NumberFormatException
可以看到Float是可以由float和double甚至string来构建的。更好的办法是使用valueOf()
函数来进行构建。
Double
的构建函数:
Double(double num)
Double(String str) throws NumberFormatException
Double支持使用double或者 str来进行构建。不过这个在JDK9里已经deprecated了,现在更多的也是使用valueOf()
。
Float
类定义的方法如下:


Double
类定义的方法如下:


这俩还包含了以下CONSTANT

下面的代码分别使用string和double来创建一个double对象
public static void main(String args[]){
Double d1 = Double.valueOf(3.14);
Double d2 = Double.valueOf("3.14");
System.out.println(d1 + "n" + d2);
理解isInfinite()以及isNan
Float和Doube都提供了isInfinite
以及isNan
方法,下面分别展示了2个无限大以及not a number的case
class RESimple{
public static void main(String args[]){
Double d1 = Double.valueOf(1d/0d);
Double d2 = Double.valueOf(0/0d);
// is infinite
System.out.println(d1);
// is not a number
System.out.println(d2);
}
}
>>>
Infinity
NaN
Byte, Short, Integer, and Long
这些包装方法和上面一样,用valueOf()
。下面贴一些这些类对应的方法
- Byte


- Short


- Integer



这俩还包含了以下2个CONSTANT

数值与字符串的相互转换: 使用xxx.parseInt()
即可
将十进制数值转换成其他位置的字符串:
public static void main(String args[]){
int num = 19648;
String bin = Integer.toBinaryString(num);
String oct = Integer.toOctalString(num);
String hex = Integer.toHexString(num);
System.out.println(bin);
System.out.println(oct);
System.out.println(hex);
>>>
100110011000000
46300
4cc0
Character
Character是char的包装类。构建方法也是valueOf();
方法,类对应的方法如下:

下面的代码展示了这个类对应的一些方法
然后我发现''
包含的是char,而""
包含的是String
class RESimple{
public static void main(String args[]){
char[] a = {'a','b','5','?','A',' '};
for(char x:a){
if (Character.isDigit(x)) System.out.println(x + " is digit");
if (Character.isLetter(x)) System.out.println(x + " is letter");
if (Character.isWhitespace(x)) System.out.println(x + " is white space");
if (Character.isLetter(x)) System.out.println(x + " is uppercase");
if (Character.isLetter(x)) System.out.println(x + " is lowercase ");
}
}
}
>>>
a is letter
a is uppercase
a is lowercase
b is letter
b is uppercase
b is lowercase
5 is digit
A is letter
A is uppercase
A is lowercase
is white space
Boolean
这个没啥好多介绍的,对应的方法如下:

Runtime
Runtime
类封装了运行环境。你不能主动启动一个Runtime
的对象。但可以对当前的Runtime
通过Runtime.getRuntime()
来得到引用。取得引用后,就可以通过一系列的方法来控制当前jvm的行为了。下面是一些示例方法:

内存管理Memory Management
虽然java 自带了内存管理,但有时你还是想知道当前对象Heap多大,还有多少内存剩余。我们可以通过使用totalMemory();
以及freeMemory()
. 另外java的垃圾回收是周期性的。有时候我们会希望根据需求来通过gc()
进行垃圾回收 。一个好的做法是先使用gc()
然后call freeMemory()
来得到内存使用的基线。然后再跑一下freeMemory
随着程序运行,来得到内存的使用情况。下面是一个示例代码:
class RESimple{
public static void main(String args[]){
Runtime r = Runtime.getRuntime();
long mem1,mem2;
Integer[] someints = new Integer[1000];
System.out.println("Total Memory is: " + r.totalMemory());
mem1 = r.freeMemory();
System.out.println("initial Memory is : " + mem1);
r.gc();
mem1 = r.freeMemory();
System.out.println("Free memory after garbage collection: " + mem1);
for(int i=0;i<1000;i++) someints[i] = null;
r.gc();
mem2 = r.freeMemory();
System.out.println("Free memory after collecting " + mem2);
}
}
>>>
Total Memory is: 270532608
initial Memory is : 268648448
Free memory after garbage collection: 9329216
Free memory after collecting 9330352
执行其他prograsms
在安全的环境中,我们可以通过不同的exec()
方法来让通过我们指定需要执行的任务名字以及参数来执行其他任务。exec()
会返回一个Process
对象用于后续控制当前进程与其他进程的交互。下面是一个在windows上跑Notepad示例:(我是mac就没跑)

返回回来的Process
可以通过waitFor()
来强制让当前进程等待。exitValue();
获取当前进程的退出码

使用currentTimeMillis()
来给程序计时
这个方法会返回当前的milliseconds时间戳
class RESimple{
public static void main(String args[]){
long start,end;
System.out.println("time for a loop from 0 to 100,000,000");
start = System.currentTimeMillis();
for(long i=0; i < 100000000L; i++);
end = System.currentTimeMillis();
System.out.println("Elapsed time : " + (end-start));
}
}
>>>
time for a loop from 0 to 100,000,000
Elapsed time : 46
用arraycopy()来拷贝数组
arraycopy()
方法可以快速的复制任何类型的数字,会比用循环快不少。
class RESimple{
public static void main(String args[]){
byte[] a = {1,2,3,4,5};
byte[] b = {5,4,3,2,1};
System.out.println(Arrays.toString(a));
System.arraycopy(a,0,b,0,a.length);
System.out.println(Arrays.toString(b));
System.out.println(a.toString());
System.out.println(b.toString());
}
}
>>>
[1, 2, 3, 4, 5]
[1, 2, 3, 4, 5]
[B@d716361
[B@6ff3c5b5
获取环境变量
我们可以通过System.getProperty()
方法来获得如下环境变量:
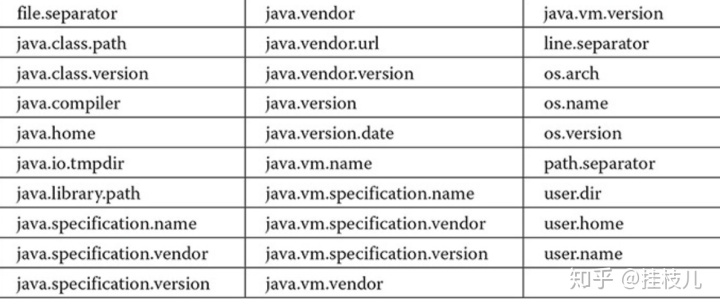
使用方法也很简单
class ShowUserDir{
public static void main(String[]){
System.out.println(System.getProperty("user.dir"));
}
}
Math库
- 三角函数:

- 反函数:

- 指数函数:

- Rounding函数:


- Miscellaneous Math Methods
Math提供了一些其他的方法。注意要以Exact
开头的那几个方法在overflow的时候会提示报错,这样子在操作运算的时候可以有效的观察到这个问题。


最后
以上就是烂漫钢笔为你收集整理的java double转float_Java完全指南 - 探索Java.lang笔记的全部内容,希望文章能够帮你解决java double转float_Java完全指南 - 探索Java.lang笔记所遇到的程序开发问题。
如果觉得靠谱客网站的内容还不错,欢迎将靠谱客网站推荐给程序员好友。
发表评论 取消回复