概述
概述
在本例中使用Apache Shiro进行授权控制,基于事先定义好的角色控制用户的操作权限。
基于Apache Shiro 提供的标签库,在JSP页面上根据用户的授权状态来控制不同的操作行为。
业务逻辑
定义两种角色:administrator,common。
administrator代表管理员,可查看,修改,删除用户;
common代表普通用户,可查看用户,只能修改自己,不能删除用户。
数据准备
创建三个表
--用户信息表
create table users(
id int NOT NULL,
name varchar(20),
passwd varchar(20),
real_name varchar(20),
sex char(1),
PRIMARY KEY (id)
);
--角色信息表
create table roles(
id int NOT NULL,
name varchar(20),
PRIMARY KEY (id)
);
--用户角色关系表
create table user_to_role(
user_id int NOT NULL,
role_id int NOT NULL,
PRIMARY KEY (user_id, role_id)
);
初始化数据
insert into users(id,name,passwd,real_name,sex) values(1,'admin','admin','管理员','1');
insert into users(id,name,passwd,real_name,sex) values(2,'Peter','Peter','彼得','1');
insert into users(id,name,passwd,real_name,sex) values(3,'Tom','Tom','汤姆','1');
insert into users(id,name,passwd,real_name,sex) values(4,'Sophie','Sophie','苏菲','0');
insert into roles(id,name) values(1,'administrator');
insert into roles(id,name) values(2,'common');
insert into user_to_role(user_id,role_id) values(1,1);
insert into user_to_role(user_id,role_id) values(2,2);
insert into user_to_role(user_id,role_id) values(3,2);
insert into user_to_role(user_id,role_id) values(4,2);
工程代码
重构抽象类AuthorizingRealm的授权方法
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principals) {
String userName = (String) principals.fromRealm(getName()).iterator().next();
//根据用户名查找拥有的角色
List<Roles> roles = userService.getUserRoles(userName);
if (roles != null) {
SimpleAuthorizationInfo info = new SimpleAuthorizationInfo();
for (Roles role : roles) {
info.addRole(role.getName());
}
return info;
} else {
return null;
}
}
展现用户信息列表Controller
@Controller
public class MainController {
private static final Logger LOGGER = LoggerFactory.getLogger(MainController.class);
@Resource(name = "userService")
private UserService userService;
@RequestMapping(value = "/main.do")
public String mainPage(HttpServletRequest request, ModelMap model) {
HttpSession session = request.getSession(true);
Subject user = SecurityUtils.getSubject();
String userID = (String) user.getPrincipal();
LOGGER.info(userID);
session.setAttribute("USERNAME", userID);
List<Users> users = userService.getAllUsers();
model.addAttribute("users", users);
return "main";
}
}
用户信息列表JSP页面
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form"%>
<%@ taglib prefix="shiro" uri="http://shiro.apache.org/tags"%>
<%
String userName = (String) session.getAttribute("USERNAME");
pageContext.setAttribute("currentUser", org.apache.shiro.SecurityUtils.getSubject().getPrincipal()
.toString());
%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Login Success</title>
<script type="text/javascript">
function confirmx(mess){
alert(mess);
}
</script>
</head>
<body bgcolor="#f3f3f3">
<p align=center>
<shiro:guest>Hi Guest</shiro:guest>
<shiro:user>
<shiro:principal />:你好,欢迎您</shiro:user>
!
</p>
<form:form>
<table id="contentTable" align=center>
<thead>
<tr>
<th>序号</th>
<th>登录名</th>
<th>姓名</th>
<th>性别</th>
<shiro:hasAnyRoles name="administrator,common">
<th>操作</th>
</shiro:hasAnyRoles>
</tr>
</thead>
<tbody>
<c:forEach items="${users}" var="user" varStatus="status">
<tr>
<td>${ status.index + 1}</td>
<td>${user.name}</td>
<td>${user.realName}</td>
<td><c:if test="${user.sex=='1'}">男</c:if>
<c:if test="${user.sex=='0'}">女</c:if></td>
<shiro:hasRole name="administrator">
<td><a href="#"
οnclick="return confirmx('确认要修改该用户吗?')">修改</a> <a
href="#" οnclick="return confirmx('确认要删除该用户吗?')">删除</a></td>
</shiro:hasRole>
<shiro:hasRole name="common">
<c:if test="${user.name==currentUser}">
<td><a href="#"
οnclick="return confirmx('确认要修改该用户吗?')">修改</a></td>
</c:if>
</shiro:hasRole>
</tr>
</c:forEach>
</tbody>
</table>
</form:form>
</body>
</html>
想使用Shiro标签首先引用标签库<%@ taglib prefix="shiro" uri="http://shiro.apache.org/tags"%>
本例中用到了<shiro:user>,<shiro:principal />,shiro:hasAnyRoles,shiro:hasRole这几个标签
效果
使用admin/admin登录,页面如下:
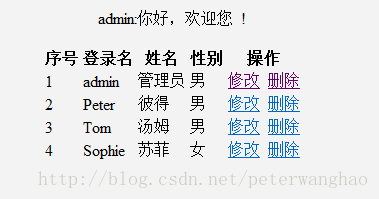
使用Peter/Peter登录,页面如下:
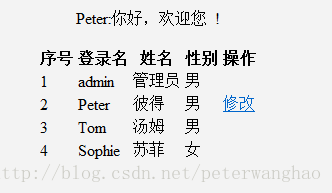
最后
以上就是有魅力手链为你收集整理的Apache Shiro 授权例子概述业务逻辑数据准备工程代码效果的全部内容,希望文章能够帮你解决Apache Shiro 授权例子概述业务逻辑数据准备工程代码效果所遇到的程序开发问题。
如果觉得靠谱客网站的内容还不错,欢迎将靠谱客网站推荐给程序员好友。
本图文内容来源于网友提供,作为学习参考使用,或来自网络收集整理,版权属于原作者所有。
发表评论 取消回复